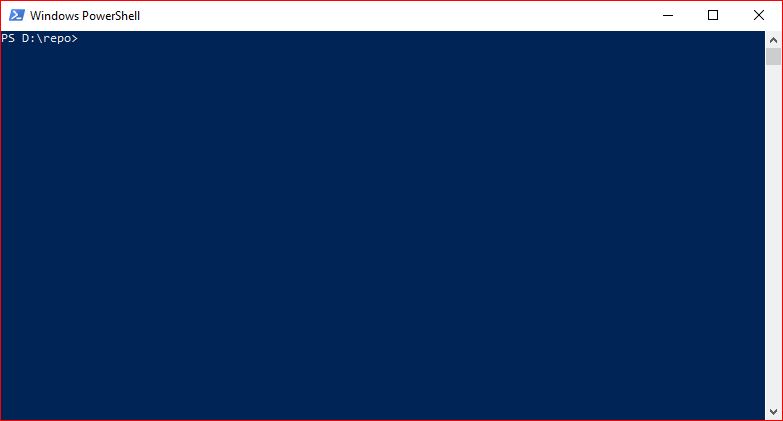
This is an old list of powershell scripts I use. I should update this list to use latest version of powershell.
Steps to create your profile
$profile
test-path $profile
#if false then create a new file for your user account
new-item -path $profile -itemtype file -force
notepad $profile
Set-ExecutionPolicy unrestricted
Create powershell functions and store them in your profile file
#Installation Step
#Install-Module posh-git -Scope CurrentUser -Force
#Install-Module oh-my-posh -Scope CurrentUser -Force
Import-Module posh-git
Import-Module oh-my-posh
Set-Theme Paradox
function _pskill ($processName="") {Get-Process | Where { $_.Name -Eq $processName } | Kill}
function _pskillMultipleProcesses ($processName="") {Get-Process $processName | Kill}
function _pskillInstance ($processName="", $windowTitleText) {Get-Process $processName | Where-Object { $_.MainWindowTitle –like ‘*'+$windowTitleText+'*’ } | Kill}
function pro { notepad $profile }
function _touch ($fileName="") {(Get-Item $fileName).lastwritetime=$(Get-Date)}
function _touch1 ($fileName="", $date) {(Get-Item $fileName).lastwritetime=$(Get-Date $date)}
function _copyToDesktop ($filename) {Copy-Item $filename ([Environment]::GetFolderPath("Desktop"))}
function _listSpecialFolders { [enum]::getvalues([system.environment+specialfolder]) | foreach {"$_ maps to " + [system.Environment]::GetFolderPath($_)}}
function _open ($pathOrFile=".") {ii $pathOrFile}
function _removeLeadingZeroes($stringValue) { $stringValue -replace '\b0+\B'}
function _tail($filename) {gc $filename -wait}
function _whoamI {[System.Security.Principal.WindowsIdentity]::GetCurrent()}
function _openWebPage($Url) { [System.Diagnostics.Process]::Start("$Url")}
function _FolderSize($Path=$home) {
$code = { ('{0:0.0} MB' -f ($this/1MB)) }
Get-ChildItem -Path $Path |
Where-Object { $_.Length -eq $null } |
ForEach-Object {
Write-Progress -Activity 'Calculating Total Size for:' -Status $_.FullName
$sum = Get-ChildItem $_.FullName -Recurse -ErrorAction SilentlyContinue |
Measure-Object -Property Length -Sum -ErrorAction SilentlyContinue
$bytes = $sum.Sum
if ($bytes -eq $null) { $bytes = 0 }
$result = 1 | Select-Object -Property Path, TotalSize
$result.Path = $_.FullName
$result.TotalSize = $bytes |
Add-Member -MemberType ScriptMethod -Name toString -Value $code -Force -PassThru
$result
}
}
function _outExcelReport
{
param ($Path = "$env:temp\$(Get-Random).csv")
$Input | Export-Csv -Path $Path -Encoding UTF8 -NoTypeInformation -UseCulture
ii -Path $Path
}
function applist { Get-WmiObject -Class Win32_Product | Select-Object -Property Name }
function EasyView { process { $_; Start-Sleep -seconds .5}}
function printGitUrls
{
$dirs = Get-ChildItem -Path . | ?{ $_.PSIsContainer }
$back = pwd
foreach ($dir in $dirs)
{
cd $dir.FullName
#echo $dir.FullName
git config --get remote.origin.url
}
cd $back.Path
}
function Talk($sentence="I am a computer")
{
Add-Type -AssemblyName System.Speech
$voice = New-Object System.Speech.Synthesis.SpeechSynthesizer
$voice.SelectVoice("Microsoft Zira Desktop")
#$voice.SelectVoice("Microsoft David Desktop")
$voice.Speak($sentence)
}
function _startNotepadWithDetachedProcess{ powershell.exe -Command "Start-Process NotePad.exe"}
function _startNotepadProcess{ $prog="C:\Windows\System32\notepad.exe"; if (! (ps | ? {$_.path -eq $prog})) { & $prog }; }
function _GitBranchesForUser($gitRepoLocation = "D://repo//hugo-arjunkrishna.us", $userName="Arjun Krishna")
{
$outputFileName = "MyBranches.txt"
cd $gitRepoLocation
$gitPruneCommand = "git remote prune origin"
bash -c $gitPruneCommand
$gitCommand = "git for-each-ref --sort=committerdate --sort=authorname --format='%(committerdate) %09 %(authorname) %09 %(refname)' | grep 'origin/'| grep '$userName' > $outputFileName"
bash -c $gitCommand
$outputFilePath = $gitRepoLocation+"//"+$outputFileName
code $outputFilePath
}
function _ShowWindows10ProductKey
{
wmic path softwarelicensingservice get OA3xOriginalProductKey
}
#Show/Email a Public Folder Calendar from your Outlook. This is helpful because when you do not have access to public folders on your mobile phone, you can schedule this to send you an email.
#_ShowPublicCalendar
#_ShowPublicCalendar -SendEmail 1
#_ShowPublicCalendar -Start '11/1/2018' -End '11/12/2018' -SendEmail 0
#_ShowPublicCalendar -Start '11/1/2018' -End '11/12/2018' -SendEmail 1
function _ShowPublicCalendar ([DateTime] $Start = [DateTime]::Now, [DateTime] $End = [DateTime]::Now.AddDays(7), $SendEmail = 1)
{
$outlook = new-object -com outlook.application
#$session = $outlook.Session
$session = $outlook.getNamespace("MAPI").Session
#$session.Logon()
$publicFolderCalendar = 18
$outlookPublicFolderRoot = $session.GetDefaultFolder($publicFolderCalendar)
$filter = "[End] >= '{0}' AND [Start] <= '{1}'" -f $Start.ToString("g"), $End.ToString("g")
$output = $outlookPublicFolderRoot.folders["SubFolderLevel1NameGoesHere"].folders["SubFolderLevel2NameGoesHere"].items.Restrict($filter) | Sort-Object Start
If ($SendEmail -eq 1)
{
$Header=@"
<style>
TABLE {border-width: 1px; border-style: solid; border-color: black; border-collapse: collapse;}
TD {border-width: 1px; padding: 3px; border-style: solid; border-color: black;}
</style>
"@
$outputHtml = $output|ConvertTo-Html -Property subject, Start, End -Head $Header
$outputString = $outputHtml | Out-String
send-mailmessage -to ak@arjunkrishna.us -from ak@arjunkrishna.us -subject 'Calendar' –body $outputString -SmtpServer mail.SmtpServerDomainNameGoesHere.com -BodyAsHtml
}
Else
{
$outputConsole = $output|Format-Table subject,Start,End -a
Write-Output $outputConsole
}
$outlook = $session = $null
}
Install powershell Core on Ubuntu 18.04
# Download the Microsoft repository GPG keys
wget -q https://packages.microsoft.com/config/ubuntu/18.04/packages-microsoft-prod.deb
# Register the Microsoft repository GPG keys
sudo dpkg -i packages-microsoft-prod.deb
# Update the list of products
sudo apt-get update
# Install PowerShell
sudo apt-get install -y powershell
# Start PowerShell
pwsh
Share this post
Twitter
Facebook
Reddit
LinkedIn
Email